Having a diagram of how your C# projects interlink, can be a big help. I've created a small PowerShell script that will produce the diagram based on the .csproj projects and their dependencies in a solution directory. It will generate yUML diagrams like this:
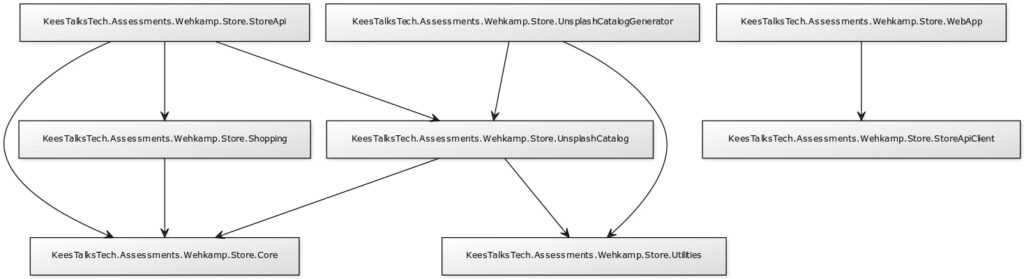
The basic idea
We're taking advantage of the fact that .csproj files for .Net Core are XML files. This means they can be queried by Select-Xml.
- Find all
.csproj
files recursively. - Exclude the test projects.
- Find all referenced project by querying the
//ProjectReference/@Include
nodes. - Map each reference (dependency) to the project by generating a
[Project]->[Reference]
string. - Join all string into a single string with the url-encoded value of
", "
=>"%2C%20"
. - Expand this string to a yuml.me URL.
- Launch the URL in the browser.
The script
The following code will open up a browser with the diagram:
(ls *.csproj -Recurse -Exclude *test* | % {
# store for later
$projectName = $_.BaseName;
# select project references
Select-Xml -Path $_.FullName -XPath "//ProjectReference/@Include" |
Select-Object -Expand Node |
Select-Object -Expand '#text' |
# extract project name for each reference
Select-String -Pattern '^(.*\\)?(.*?)(.csproj)?$' |
% { $_.Matches.Groups[2].Value } |
# create the yUML reference
% { "[" + $projectName + "]->[" + $_ + "]" }
}) -join "%2C%20" | # join using ", " URL encoded string
% { start -filepath ('http://yuml.me/diagram/plain/class/' + $_) }
Pretty cool, right? Thanks to the guys of yuml.me!
Listing Nuget packagess
If you like to create a diagram of both project and Nuget packages, you can use the following script (the Select-Xml statement is now changed). Note: these diagrams are very large. Some URLs are way too big to start. Handle with care!
(ls *.csproj -Recurse -Exclude *test* | % {
# store for later
$projectName = $_.BaseName;
# select project references
Select-Xml -Path $_.FullName -XPath "//*[self::ProjectReference or self::PackageReference]/@Include" |
Select-Object -Expand Node |
Select-Object -Expand '#text' |
# extract project name for each reference
Select-String -Pattern '^(.*\\)?(.*?)(.csproj)?$' |
% { $_.Matches.Groups[2].Value } |
# create the yUML reference
% { "[" + $projectName + "]->[" + $_ + "]" }
}) -join "%2C%20" | # join using ", " URL encoded string
% { start -filepath ('http://yuml.me/diagram/plain/class/' + $_) }
The diagram will be way bigger:
The URL that the script generates is: huuuuuuuuuge!
Pretty cool, right? Thanks to the guys of yuml.me!